共计 4186 个字符,预计需要花费 11 分钟才能阅读完成。
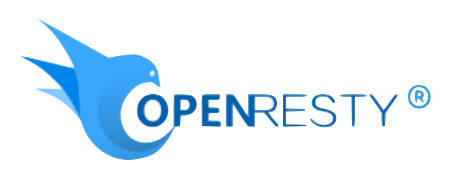
一个简单的计算api服务,提供:加减乘除,从这个示例可以get到:
- 用table来创建module
- 用require来导入module
- 把lua代码放入各自lua文件中并调用
- 设置lua搜索路径
- 校验参数
模块创建和引入
用table来创建module
在lua中使用table来创建一个module
[root@nginx-cluster lua]# cat my.lua
local _M = {}
local function get_name()
return ""
end
function _M.greeting()
print("hello " .. get_name())
end
return _M
用require来导入module
[root@nginx-cluster lua]# cat main.lua
local my_module = require("my")
my_module.greeting()
测试运行
[root@nginx-cluster lua]# luajit main.lua
hello Xadocker
设置lua搜索路径
而在openresty中使用时,需要定义lua的搜索路径,不然会提示找不到模块
2020/04/14 20:37:23 [error] 24930#0: *1 lua entry thread aborted: runtime error: /usr/local/openresty/nginx/lua/access_check.lua:1: module 'comm.param' not found:
所以我们需要在openresty中创建一个目录用来专门放置lua处理脚本,我们把它创建在openresty的prefix内,这样方便配置文件的移植
# 查看当前openresty的prefix
[root@nginx-cluster lua]# openresty -V
nginx version: openresty/1.17.8.2
built by gcc 4.8.5 20150623 (Red Hat 4.8.5-44) (GCC)
built with OpenSSL 1.0.2k-fips 26 Jan 2017
TLS SNI support enabled
configure arguments: --prefix=/usr/local/openresty/nginx --with-cc-opt=-O2 --add-module=../ngx_devel_kit-0.3.1 --add-module=../echo-nginx-module-0.62 --add-module=../xss-nginx-module-0.06 --add-module=../ngx_coolkit-0.2 --add-module=../set-misc-nginx-module-0.32 --add-module=../form-input-nginx-module-0.12 --add-module=../encrypted-session-nginx-module-0.08 --add-module=../srcache-nginx-module-0.32 --add-module=../ngx_lua-0.10.17 --add-module=../ngx_lua_upstream-0.07 --add-module=../headers-more-nginx-module-0.33 --add-module=../array-var-nginx-module-0.05 --add-module=../memc-nginx-module-0.19 --add-module=../redis2-nginx-module-0.15 --add-module=../redis-nginx-module-0.3.7 --add-module=../rds-json-nginx-module-0.15 --add-module=../rds-csv-nginx-module-0.09 --add-module=../ngx_stream_lua-0.0.8 --with-ld-opt=-Wl,-rpath,/usr/local/openresty/luajit/lib --with-stream --with-stream_ssl_module --with-stream_ssl_preread_module --with-http_ssl_module
# 可以看到此时openresty的prefix是/usr/local/openresty/nginx
# 创建lua脚本放置路径
[root@nginx-cluster ~]# mkdir /usr/local/openresty/nginx/lua
在nginx主配置文件http块内定义lua搜索路径
http {
.....略.....
lua_package_path '$prefix/lua/?.lua;/blah/?.lua;;';
include conf.d/*.conf;
}
在access阶段校验参数
access_by_lua*:在access阶段对参数进行校验,此处逻辑写入lua/access_check.lua。文件内容为:
[root@nginx-cluster ~]# cat /usr/local/openresty/nginx/lua/access_check.lua
--- 导入模块,会从定义的lua搜索路径中进行查找
local param= require("comm.param")
--- 获取get请求参数
local args = ngx.req.get_uri_args()
if not args.a or not args.b or not param.is_number(args.a, args.b) then
ngx.exit(ngx.HTTP_BAD_REQUEST)
return
end
comm.param对应的模块文件内容
[root@nginx-cluster ~]# cat /usr/local/openresty/nginx/lua/comm/param.lua
local _M = {}
function _M.is_number(...)
local arg = {...}
local num
for _,v in ipairs(arg) do
num = tonumber(v)
if nil == num then
return false
end
end
return true
end
return _M
在content阶段从lua脚本中处理响应
content_by_lua*:在content阶段进行内容输出,此处加减乘除共四个分别写入到各自文件中,其内容为
[root@nginx-cluster ~]# cat /usr/local/openresty/nginx/lua/simple_cal.lua
local args = ngx.req.get_uri_args()
ngx.say(args.a + args.b)
[root@nginx-cluster ~]# cat /usr/local/openresty/nginx/lua/subtraction.lua
local args = ngx.req.get_uri_args()
ngx.say(args.a - args.b)
[root@nginx-cluster ~]# cat /usr/local/openresty/nginx/lua/multiplication.lua
local args = ngx.req.get_uri_args()
ngx.say(args.a * args.b)
[root@nginx-cluster ~]# cat /usr/local/openresty/nginx/lua/division.lua
local args = ngx.req.get_uri_args()
ngx.say(args.a / args.b)
测试服务
最后我们创建简单计算服务的nginx server配置
server {
listen 80;
server_name simple-cal.xadocker.cn;
location ~ ^/api/([-_a-zA-Z0-9/]+) {
access_by_lua_file lua/access_check.lua;
content_by_lua_file lua/$1.lua;
}
}
重载openresty服务
[root@nginx-cluster ~]# openresty -t
nginx: the configuration file /usr/local/openresty/nginx/conf/nginx.conf syntax is ok
nginx: configuration file /usr/local/openresty/nginx/conf/nginx.conf test is successful
[root@nginx-cluster ~]# openresty -s reload
测试服务运行
[root@nginx-cluster lua]# curl 'simple-cal.xadocker.cn/api/addition?a=1&b=3'
4
[root@nginx-cluster lua]# curl 'simple-cal.xadocker.cn/api/subtraction?a=1&b=3'
-2
[root@nginx-cluster lua]# curl 'simple-cal.xadocker.cn/api/multiplication?a=2&b=3'
6
[root@nginx-cluster lua]# curl 'simple-cal.xadocker.cn/api/division?a=2&b=3'
0.66666666666667
[root@nginx-cluster lua]# curl 'simple-cal.xadocker.cn/api/addition?a=b&b=3'
<html>
<head><title>400 Bad Request</title></head>
<body>
<center><h1>400 Bad Request</h1></center>
<hr><center>openresty/1.17.8.2</center>
</body>
</html>
正文完